2019-05-02 18:17:27 -04:00
|
|
|
# frozen_string_literal: true
|
|
|
|
|
2013-02-27 22:39:42 -05:00
|
|
|
class Admin::ReportsController < Admin::AdminController
|
2018-06-18 06:31:56 -04:00
|
|
|
def index
|
2018-11-19 06:20:05 -05:00
|
|
|
reports_methods = ['page_view_total_reqs'] +
|
|
|
|
ApplicationRequest.req_types.keys
|
|
|
|
.select { |r| r =~ /^page_view_/ && r !~ /mobile/ }
|
|
|
|
.map { |r| r + "_reqs" } +
|
2018-12-27 16:21:08 -05:00
|
|
|
Report.singleton_methods.grep(/^report_(?!about|storage_stats)/)
|
2018-06-18 06:31:56 -04:00
|
|
|
|
|
|
|
reports = reports_methods.map do |name|
|
|
|
|
type = name.to_s.gsub('report_', '')
|
|
|
|
description = I18n.t("reports.#{type}.description", default: '')
|
2020-03-02 14:30:51 -05:00
|
|
|
description_link = I18n.t("reports.#{type}.description_link", default: '')
|
2018-06-18 06:31:56 -04:00
|
|
|
|
|
|
|
{
|
|
|
|
type: type,
|
|
|
|
title: I18n.t("reports.#{type}.title"),
|
|
|
|
description: description.presence ? description : nil,
|
2020-03-02 14:30:51 -05:00
|
|
|
description_link: description_link.presence ? description_link : nil
|
2018-06-18 06:31:56 -04:00
|
|
|
}
|
|
|
|
end
|
|
|
|
|
|
|
|
render_json_dump(reports: reports.sort_by { |report| report[:title] })
|
|
|
|
end
|
2013-02-27 22:39:42 -05:00
|
|
|
|
2018-08-24 09:28:01 -04:00
|
|
|
def bulk
|
|
|
|
reports = []
|
2013-02-27 22:39:42 -05:00
|
|
|
|
2018-08-24 09:28:01 -04:00
|
|
|
hijack do
|
|
|
|
params[:reports].each do |report_type, report_params|
|
|
|
|
args = parse_params(report_params)
|
2013-02-27 22:39:42 -05:00
|
|
|
|
2018-08-24 09:28:01 -04:00
|
|
|
report = nil
|
|
|
|
if (report_params[:cache])
|
|
|
|
report = Report.find_cached(report_type, args)
|
|
|
|
end
|
2017-04-13 05:10:55 -04:00
|
|
|
|
2018-08-24 09:28:01 -04:00
|
|
|
if report
|
|
|
|
reports << report
|
|
|
|
else
|
|
|
|
report = Report.find(report_type, args)
|
2015-06-24 09:19:39 -04:00
|
|
|
|
2018-08-24 09:28:01 -04:00
|
|
|
if (report_params[:cache]) && report
|
2020-12-09 11:54:41 -05:00
|
|
|
Report.cache(report)
|
2018-08-24 09:28:01 -04:00
|
|
|
end
|
2016-02-02 21:29:51 -05:00
|
|
|
|
2018-11-12 07:47:24 -05:00
|
|
|
if report.blank?
|
|
|
|
report = Report._get(report_type, args)
|
|
|
|
report.error = :not_found
|
|
|
|
end
|
|
|
|
|
|
|
|
reports << report
|
2018-08-24 09:28:01 -04:00
|
|
|
end
|
|
|
|
end
|
2018-05-10 23:30:21 -04:00
|
|
|
|
2018-08-24 09:28:01 -04:00
|
|
|
render_json_dump(reports: reports)
|
2018-05-15 01:08:23 -04:00
|
|
|
end
|
2018-08-24 09:28:01 -04:00
|
|
|
end
|
2018-05-15 01:08:23 -04:00
|
|
|
|
2018-08-24 09:28:01 -04:00
|
|
|
def show
|
|
|
|
report_type = params[:type]
|
|
|
|
|
|
|
|
raise Discourse::NotFound unless report_type =~ /^[a-z0-9\_]+$/
|
|
|
|
|
|
|
|
args = parse_params(params)
|
2018-05-16 02:05:03 -04:00
|
|
|
|
|
|
|
report = nil
|
|
|
|
if (params[:cache])
|
|
|
|
report = Report.find_cached(report_type, args)
|
|
|
|
end
|
|
|
|
|
|
|
|
if report
|
|
|
|
return render_json_dump(report: report)
|
|
|
|
end
|
|
|
|
|
|
|
|
hijack do
|
|
|
|
report = Report.find(report_type, args)
|
|
|
|
|
|
|
|
raise Discourse::NotFound if report.blank?
|
|
|
|
|
|
|
|
if (params[:cache])
|
2020-12-09 11:54:41 -05:00
|
|
|
Report.cache(report)
|
2018-05-16 02:05:03 -04:00
|
|
|
end
|
|
|
|
|
|
|
|
render_json_dump(report: report)
|
|
|
|
end
|
2013-02-27 22:39:42 -05:00
|
|
|
end
|
|
|
|
|
2018-08-24 09:28:01 -04:00
|
|
|
private
|
|
|
|
|
|
|
|
def parse_params(report_params)
|
2020-01-03 09:01:38 -05:00
|
|
|
begin
|
|
|
|
start_date = (report_params[:start_date].present? ? Time.parse(report_params[:start_date]).to_date : 1.days.ago).beginning_of_day
|
|
|
|
end_date = (report_params[:end_date].present? ? Time.parse(report_params[:end_date]).to_date : start_date + 30.days).end_of_day
|
|
|
|
rescue ArgumentError => e
|
|
|
|
raise Discourse::InvalidParameters.new(e.message)
|
|
|
|
end
|
2018-08-24 09:28:01 -04:00
|
|
|
|
|
|
|
facets = nil
|
|
|
|
if Array === report_params[:facets]
|
|
|
|
facets = report_params[:facets].map { |s| s.to_s.to_sym }
|
|
|
|
end
|
|
|
|
|
|
|
|
limit = nil
|
|
|
|
if report_params.has_key?(:limit) && report_params[:limit].to_i > 0
|
|
|
|
limit = report_params[:limit].to_i
|
|
|
|
end
|
|
|
|
|
2019-04-26 06:17:10 -04:00
|
|
|
filters = nil
|
|
|
|
if report_params.has_key?(:filters)
|
|
|
|
filters = report_params[:filters]
|
FEATURE: Exposing a way to add a generic report filter (#6816)
* FEATURE: Exposing a way to add a generic report filter
## Why do we need this change?
Part of the work discussed [here](https://meta.discourse.org/t/gain-understanding-of-file-uploads-usage/104994), and implemented a first spike [here](https://github.com/discourse/discourse/pull/6809), I am trying to expose a single generic filter selector per report.
## How does this work?
We basically expose a simple, single generic filter that is computed and displayed based on backend values passed into the report.
This would be a simple contract between the frontend and the backend.
**Backend changes:** we simply need to return a list of dropdown / select options, and enable the report's newly introduced `custom_filtering` property.
For example, for our [Top Uploads](https://github.com/discourse/discourse/pull/6809/files#diff-3f97cbb8726f3310e0b0c386dbe89e22R1423) report, it can look like this on the backend:
```ruby
report.custom_filtering = true
report.custom_filter_options = [{ id: "any", name: "Any" }, { id: "jpg", name: "JPEG" } ]
```
In our javascript report HTTP call, it will look like:
```js
{
"custom_filtering": true,
"custom_filter_options": [
{
"id": "any",
"name": "Any"
},
{
"id": "jpg",
"name": "JPG"
}
]
}
```
**Frontend changes:** We introduced a generic `filter` param and a `combo-box` which hooks up into the existing framework for fetching a report.
This works alright, with the limitation of being a single custom filter per report. If we wanted to add, for an instance a `filesize filter`, this will not work for us. _I went through with this approach because it is hard to predict and build abstractions for requirements or problems we don't have yet, or might not have._
## How does it look like?
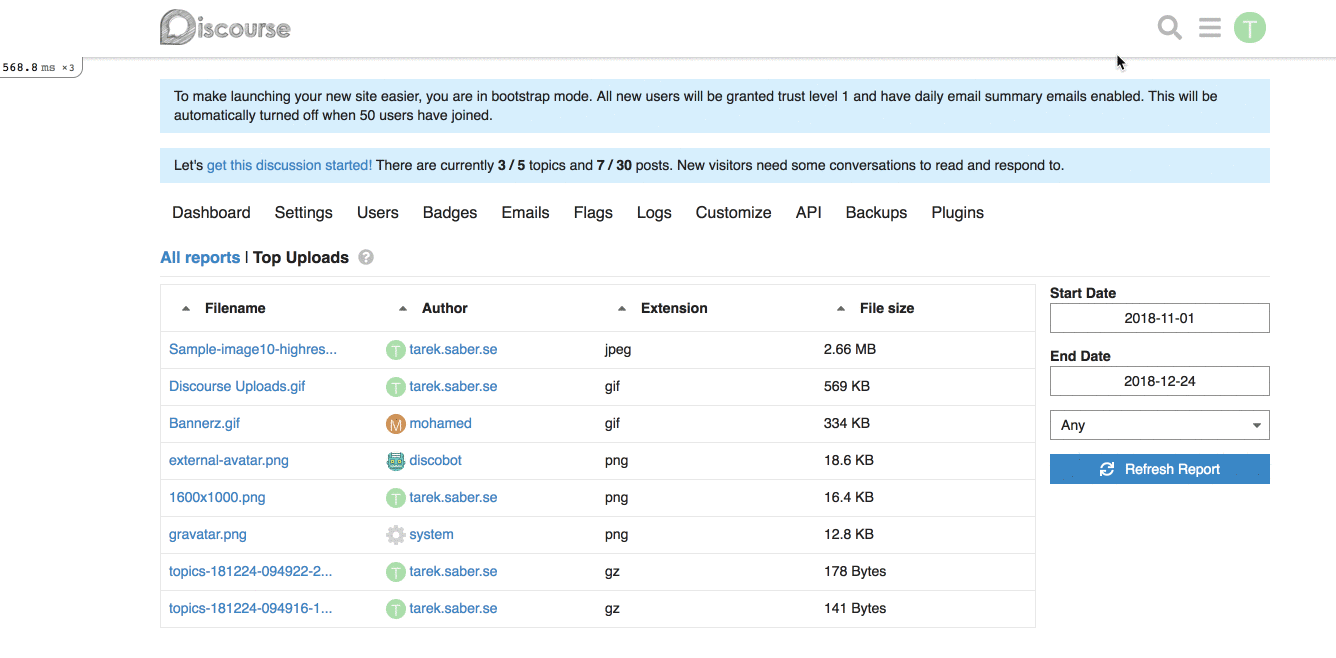
## More on the bigger picture
The major concern here I have is the solution I introduced might serve the `think small` version of the reporting work, but I don't think it serves the `think big`, I will try to shed some light into why.
Within the current design, It is hard to maintain QueryParams for dynamically generated params (based on the idea of introducing more than one custom filter per report).
To allow ourselves to have more than one generic filter, we will need to:
a. Use the Route's model to retrieve the report's payload (we are now dependent on changes of the QueryParams via computed properties)
b. After retrieving the payload, we can use the `setupController` to define our dynamic QueryParams based on the custom filters definitions we received from the backend
c. Load a custom filter specific Ember component based on the definitions we received from the backend
2019-03-15 08:15:38 -04:00
|
|
|
end
|
|
|
|
|
2018-08-24 09:28:01 -04:00
|
|
|
{
|
|
|
|
start_date: start_date,
|
|
|
|
end_date: end_date,
|
2019-04-26 06:17:10 -04:00
|
|
|
filters: filters,
|
2018-08-24 09:28:01 -04:00
|
|
|
facets: facets,
|
|
|
|
limit: limit
|
|
|
|
}
|
|
|
|
end
|
2013-02-27 22:39:42 -05:00
|
|
|
end
|